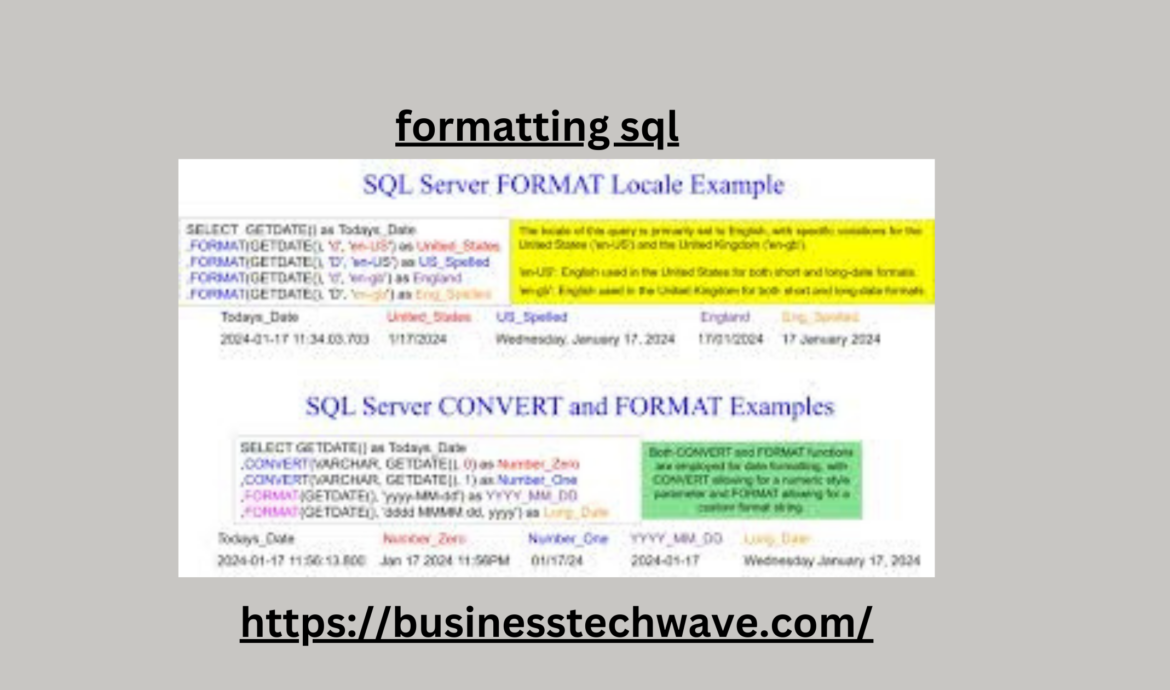
Mastering Formatting SQL: Best Practices and Tips 2024
Ramzanmughal103@gmail.com October 22, 2024 ArticleWhen it comes to writing SQL queries, clarity is key. Properly formatting SQL is essential for anyone working with databases. Whether you are a beginner or an experienced developer, the way you structure and present your SQL code impacts both readability and maintainability. This article covers essential guidelines and best practices for formatting SQL to make your queries cleaner, more understandable, and more efficient.
Why is Formatting SQL Important?
Formatting SQL is not just about aesthetics; it’s about functionality and efficiency. Here are some reasons why formatting SQL is crucial:
- Readability: Well-formatted SQL is easier to read, understand, and debug. It allows developers to quickly comprehend what a query is doing.
- Maintainability: Proper formatting helps when revisiting SQL code in the future. It’s easier to identify issues or make updates when the SQL is well-structured.
- Collaboration: In team environments, consistent formatting ensures that every team member can understand and contribute to the SQL code without ambiguity.
- Error Prevention: Properly formatted SQL reduces the risk of syntax errors, making it easier to spot mistakes before execution.
SQL Formatting Best Practices
To ensure that your SQL code is well-formatted, here are some best practices you should follow:

1. Use Uppercase for SQL Keywords
One of the simplest rules for formatting SQL is to use uppercase letters for all SQL keywords such as SELECT
, FROM
, WHERE
, JOIN
, and GROUP BY
. This makes the commands stand out clearly from table names and column names, improving readability.
Example:
sqlCopy codeSELECT first_name, last_name
FROM employees
WHERE department = 'Sales'
ORDER BY hire_date DESC;
2. Align SQL Code Vertically
When writing SQL code, aligning similar elements vertically improves clarity. Consider breaking lines at logical points and keeping similar keywords aligned. This makes the query structure apparent at a glance.
Example:
sqlCopy codeSELECT
first_name,
last_name,
department,
hire_date
FROM
employees
WHERE
department = 'Marketing'
AND hire_date > '2022-01-01'
ORDER BY
hire_date DESC;
3. Use Indentation for Nested Queries
Indentation plays a vital role in formatting SQL, especially when dealing with subqueries. Indent nested queries to make them visually distinct from the main query. This makes it easier to follow the logical flow of the query.
Example:
sqlCopy codeSELECT department, AVG(salary)
FROM (
SELECT
department,
salary
FROM
employees
WHERE
hire_date > '2021-01-01'
) AS recent_hires
GROUP BY department;
4. Use Meaningful Aliases
Aliases help simplify long table and column names, but it’s important to use aliases that are meaningful and easy to understand. Always choose an alias that makes sense in the context of your query.
Example:
sqlCopy codeSELECT
e.first_name AS emp_first,
e.last_name AS emp_last,
d.department_name AS dept_name
FROM
employees AS e
JOIN
departments AS d
ON
e.department_id = d.department_id;
5. Avoid Long Lines
Long lines can make your SQL code difficult to read, especially on smaller screens. Instead, break long lines into multiple shorter lines, aligning them for better readability.
Example:
sqlCopy codeSELECT
product_name,
product_category,
price,
stock_quantity,
supplier_id
FROM
products
WHERE
product_category = 'Electronics'
AND stock_quantity > 10
AND price BETWEEN 100 AND 500
ORDER BY
price DESC;
Advanced SQL Formatting Techniques
Once you are familiar with the basics, you can move on to advanced formatting techniques that involve more complex SQL scenarios.
6. Formatting SQL Joins
SQL JOIN
statements can become complex, especially with multiple conditions. Proper formatting of JOIN
clauses makes it clear which tables are being joined and what conditions are being used. Each JOIN
should be on a new line, and conditions should be clearly aligned.
Example:
sqlCopy codeSELECT
c.customer_name,
o.order_date,
p.product_name,
p.price
FROM
customers AS c
INNER JOIN
orders AS o
ON
c.customer_id = o.customer_id
LEFT JOIN
products AS p
ON
o.product_id = p.product_id
WHERE
o.order_date > '2023-01-01'
ORDER BY
o.order_date DESC;
7. Organize GROUP BY
and ORDER BY
Clauses
Always place GROUP BY
and ORDER BY
clauses on new lines. It’s best to list the columns used for grouping or ordering vertically to maintain readability, especially when there are multiple columns.
Example:
sqlCopy codeSELECT
department,
COUNT(*) AS total_employees,
AVG(salary) AS average_salary
FROM
employees
GROUP BY
department
ORDER BY
average_salary DESC;
8. Keep Complex Conditions Clear
When dealing with complex WHERE
conditions, it’s best to format them in a way that makes the logical operators (AND
, OR
) clearly visible. Each condition should be on its own line for better visibility.
Example:
sqlCopy codeSELECT
product_name,
price,
category
FROM
products
WHERE
category = 'Appliances'
AND (price > 1000 OR stock_quantity < 5)
AND supplier_id IN (101, 102, 103);
Common Mistakes to Avoid When Formatting SQL
9. Avoid Using SELECT *
While it’s tempting to use SELECT *
for quick queries, it’s not a good practice for production environments. Always specify the columns you need. This improves readability and performance by only retrieving necessary data.
Example:
sqlCopy code-- Avoid this:
SELECT * FROM employees;
-- Use this instead:
SELECT first_name, last_name, department FROM employees;
10. Do Not Nest Too Many Subqueries
Over-nesting subqueries can make SQL queries confusing and hard to debug. Try to keep your SQL flat when possible, using JOIN
or WITH
(Common Table Expressions) instead of deep nesting.
Example using CTE:
sqlCopy codeWITH recent_employees AS (
SELECT
first_name,
last_name,
hire_date
FROM
employees
WHERE
hire_date > '2022-01-01'
)
SELECT * FROM recent_employees WHERE hire_date > '2023-01-01';
11. Use Consistent Naming Conventions
Adopt a consistent naming convention for tables, columns, and aliases. Whether you use camelCase, snake_case, or another format, consistency is key to formatting SQL effectively. Stick to your chosen convention throughout your database code.
Tools to Help with SQL Formatting
Several tools can automatically format SQL, making it easy to adhere to best practices. Some popular SQL formatting tools include:
- SQL Formatter Online: A web-based tool for quickly formatting SQL.
- SQL Server Management Studio (SSMS): Has built-in SQL formatting features.
- DBeaver: An open-source database tool with SQL formatting support.
- DataGrip: A database IDE by JetBrains that offers robust SQL formatting options.
These tools allow you to format SQL consistently, saving you time and ensuring adherence to standards.
SQL Formatting Checklist
To summarize the best practices, here’s a quick checklist you can use for formatting SQL:
- Use uppercase for SQL keywords.
- Align similar elements vertically.
- Indent nested queries.
- Use meaningful and concise aliases.
- Avoid excessively long lines.
- Format
JOIN
clauses on new lines. - Use consistent naming conventions.
- Avoid using
SELECT *
; specify columns instead. - Keep
WHERE
conditions and logical operators clear. - Use tools for consistent SQL formatting.
Conclusion
Formatting SQL is a skill that every database developer should master. It goes beyond making your SQL queries look good; it’s about writing code that is easy to read, maintain, and debug. Whether you are working solo or in a team, following proper SQL formatting guidelines will help you produce cleaner, more efficient, and maintainable database code. Take the time to learn and apply these best practices in your SQL projects.
By incorporating these strategies, you will enhance your SQL code’s quality, making it easier to manage in the long run. Remember, formatting SQL is not just about following rules—it’s about creating a clear, logical, and efficient way to interact with your data.
Leave a Reply